For loops are an important part of Java programming and are used to execute a block of code repeatedly. They are especially useful when working with arrays or collections, allowing you to easily access and manipulate each element in the array or collection.
In this tutorial, you will learn about the syntax of a for loop, using a for loop to iterate over arrays or collections, nested for loops, and using break and continue statements. Additionally, you will also learn the forEach method, a powerful and efficient way to iterate over arrays or collections introduced in Java 8. By the end of this tutorial, you will have a solid understanding of how to use for loops and the forEach method in your own Java programs.
What are loops in Java?
Loops in Java are used to repeat code a controlled number of times. The number of times the code block is repeated is controlled by a logical expression. The code block will continue to be repeated as long as the logical expression evaluates to true, and it will stop as soon as the logical expression becomes false.
There are three types of loops in Java:
- the for loop – is used when you know the exact number of times you want the loop to execute.
- the while loop – is used when you don’t know the exact number of times the loop should execute, but you do know the condition that should cause the loop to stop.
- the do-while loop – is similar to the while loop, but it guarantees that the loop will execute at least once.
In this tutorial, I’ll be focusing on the for loop because it’s a common and versatile loop structure that’s used in many Java programs.
Java for loop
In Java, a for loop is a control structure that allows you to repeatedly execute a block of code a certain number of times. The basic syntax for a for loop is:
for (initialization; condition; increment/decrement) { // code block to be executed }
-
The
for
keyword is used to indicate that a for loop is being used. -
The
initialization
component is used to set the initial value of a loop control variable. This is typically a counter variable that will be used to keep track of how many times the loop has executed. -
The
condition
component is a boolean expression that is evaluated before each iteration of the loop. If the expression evaluates totrue
, the loop continues to execute. If it evaluates tofalse
, the loop terminates. -
The
increment/decrement
component is used to modify the loop control variable after each iteration of the loop. This can be an increment (adding 1 to the variable) or a decrement (subtracting 1 from the variable). -
The code block to be executed is enclosed in curly braces
{}
and is executed repeatedly as long as the condition evaluates totrue
.
The flow of the for loop
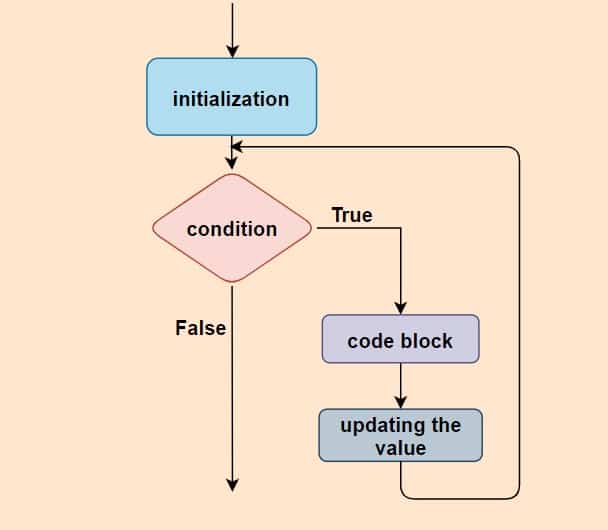
Understanding the flow of a for loop is important to know how the code within the loop executes. Here’s the step-by-step flow of a for loop in Java:
-
The
initialization
is executed first and only once at the beginning of the loop. This is where you set the loop control variable to an initial value. -
Next, the
condition
is evaluated. If the condition evaluates totrue
, the code block is executed. If the condition evaluates tofalse
, the loop is terminated and the program continues to execute after the loop. -
The code block within the loop is executed.
-
After the code block is executed, the
increment/decrement
is executed. This is where the loop control variable is updated. -
Then, the
condition
is evaluated again. If the condition still evaluates totrue
, the loop continues to execute from step 3. If the condition evaluates tofalse
, the loop is terminated and the program continues to execute after the loop. -
The loop continues to execute in this manner until the
condition
evaluates tofalse
.
Here’s an example to illustrate the flow of a for loop:
for (int i = 0; i < 5; i++) { System.out.println("Iteration " + (i+1)); }
In this example:
-
The
initialization
sets the loop control variablei
to 0. -
The
condition
checks whetheri
is less than 5. Sincei
is 0, the condition istrue
and the loop begins. -
The code block prints “Iteration 1” to the console.
-
The
increment/decrement
incrementsi
by 1, making it 1. -
The
condition
checks whetheri
is less than 5. Sincei
is 1, the condition istrue
, and the loop continues. -
The code block prints “Iteration 2” to the console.
-
The
increment/decrement
incrementsi
by 1, making it 2. -
The
condition
checks whetheri
is less than 5. Sincei
is 2, the condition istrue
, and the loop continues. -
The code block prints “Iteration 3” to the console.
-
The
increment/decrement
incrementsi
by 1, making it 3. -
The
condition
checks whetheri
is less than 5. Sincei
is 3, the condition istrue
, and the loop continues. -
The code block prints “Iteration 4” to the console.
-
The
increment/decrement
incrementsi
by 1, making it 4. -
The
condition
checks whetheri
is less than 5. Sincei
is 4, the condition istrue
, and the loop continues. -
The code block prints “Iteration 5” to the console.
-
The
increment/decrement
incrementsi
by 1, making it 5. -
The
condition
checks whetheri
is less than 5. Sincei
is now 5, the condition isfalse
, and the loop terminates.
The output of this loop would be:
Iteration 1 Iteration 2 Iteration 3 Iteration 4 Iteration 5
In summary, the syntax of a for loop in Java consists of four components: initialization, condition, increment/decrement, and code block. The initialization sets the loop control variable, the condition checks whether the loop should continue, the increment/decrement modifies the loop control variable, and the code block is executed repeatedly as long as the condition is true.
Using a for loop to iterate over an array or collection
One of the most common uses of a for loop in Java is to iterate over each element in an array or collection. Here’s how to use a for loop to access and manipulate array or collection elements:
Iterating over an array
To iterate over an array using a for loop, you’ll need to know the length of the array. You can get the length of an array using the .length property. Here’s an example:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { System.out.println(numbers[i]); }
In this example, we create an array called numbers
with five elements. We then use a for loop to iterate over each element in the array. The loop starts at index 0 (the first element in the array) and continues until it reaches the length of the array. Inside the loop, we print out each element in the array using the index variable i
.
Iterating over a collection
To iterate over a collection using a for loop, you can use the enhanced for loop syntax (also known as the “for-each” loop). Here’s an example:
List<String> names = new ArrayList<String>(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); for (String name : names) { System.out.println(name); }
In this example, we create an ArrayList called names
with three elements. We then use a for-each loop to iterate over each element in the list. Inside the loop, we print out each element using the loop variable name
.
Note that when using a for-each loop, you don’t need to know the size of the collection. The loop will automatically iterate over each element in the collection.
Manipulating array or collection elements
You can also use a for loop to access and manipulate each element in an array or collection. Here’s an example:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { numbers[i] = numbers[i] * 2; } for (int number : numbers) { System.out.println(number); }
In this example, we create an array called numbers
with five elements. We then use a for loop to iterate over each element in the array and multiply it by 2. Finally, we use a for-each loop to print out each element in the modified array.
By using a for loop to iterate over an array or collection, you can easily access and manipulate each element in the collection. This makes for loops a powerful tool for many types of programming tasks.
Nested for loops
In Java, it’s possible to use a for loop inside another for loop, creating a nested loop structure. This can be useful for iterating over multiple arrays or collections simultaneously or for performing a set of operations multiple times based on some conditions. So, a nested loop in Java is simply a loop inside another loop.
The basic syntax for a nested for loop is as follows:
for (int i = 0; i < numRows; i++) { for (int j = 0; j < numCols; j++) { // Perform some operations here } }
In this example, we have an outer loop that iterates over a certain number of rows, and an inner loop that iterates over a certain number of columns. For each row, the inner loop will execute its operations a certain number of times based on the number of columns.
Nested loops can be especially useful when working with multi-dimensional arrays or collections. For example, let’s say we have a 2D array representing a tic-tac-toe board, and we want to check if there is a winning combination of X’s or O’s. We could use nested loops to check each row, column, and diagonal:
char[][] board = { {'X', 'O', 'X'}, {'O', 'X', 'O'}, {'X', 'O', 'X'} }; // Check rows for (int i = 0; i < 3; i++) { boolean hasWon = true; for (int j = 0; j < 3; j++) { if (board[i][j] != 'X') { hasWon = false; break; } } if (hasWon) { System.out.println("X has won!"); break; } } // Check columns for (int j = 0; j < 3; j++) { boolean hasWon = true; for (int i = 0; i < 3; i++) { if (board[i][j] != 'O') { hasWon = false; break; } } if (hasWon) { System.out.println("O has won!"); break; } } // Check diagonals boolean hasWon = true; for (int i = 0; i < 3; i++) { if (board[i][i] != 'X') { hasWon = false; break; } } if (hasWon) { System.out.println("X has won!"); } else { hasWon = true; for (int i = 0; i < 3; i++) { if (board[i][2-i] != 'O') { hasWon = false; break; } } if (hasWon) { System.out.println("O has won!"); } }
In this example, we use nested loops to check each row, column, and diagonal for a winning combination of X’s or O’s. The outer loop iterates over the rows or columns, and the inner loop iterates over the cells within each row or column.
It’s important to be careful when using nested loops, as they can quickly become complex and difficult to read. It’s a good idea to keep the number of nested loops to a minimum, and to use clear and descriptive variable names to help make the code more readable.
Using a for loop with break and continue statements
In addition to using a for loop to iterate over elements in an array or collection, you can also use two statements – break
and continue
– to control the flow of the loop.
The break
statement allows you to exit the loop early if a certain condition is met. When the break
statement is executed, the loop will terminate immediately and control will pass to the statement following the loop.
On the other hand, the continue
statement allows you to skip over certain iterations of the loop if a certain condition is met. When the continue
statement is executed, the loop will immediately move on to the next iteration, skipping over any code that comes after the continue
statement in the current iteration.
For example, consider the following code that uses a for loop to iterate over an array and print out each element:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { if (numbers[i] == 3) { break; // exit the loop if the current element is 3 } System.out.println(numbers[i]); }
In this example, the loop will terminate immediately when it encounters the element with value 3
, and will not print out the remaining elements of the array.
To learn more about how to use the break
and continue
statements in Java, you can check out this tutorial How to break out of the loop in Java?, it provides more in-depth explanations and examples of how to use these statements effectively in your Java code.
Java forEach method
In addition to the traditional for loop, Java also provides the forEach method for iterating over arrays or collections. The forEach method was introduced in Java 8 and provides a concise and efficient way to iterate over elements in a collection or array.
Syntax of forEach Method
The syntax of the forEach method is as follows:
collectionOrArray.forEach(element -> { // code to be executed for each element });
In this syntax, collectionOrArray
is the collection or array to be iterated over, and element
represents each element in the collection or array. The code within the curly braces {}
is the code that will be executed for each element.
Example Usage of forEach Method
Here is an example of using the forEach method to iterate over an ArrayList of strings and print each string to the console:
import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> names = new ArrayList<String>(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); names.forEach(name -> { System.out.println(name); }); } }
Output:
Alice Bob Charlie
In this example, we first create an ArrayList of strings called names
. We then add three strings to this ArrayList. Finally, we use the forEach method to iterate over each string in the names
ArrayList and print it to the console.
Advantages of forEach Method
The forEach method has several advantages over the traditional for loop. First, it provides a more concise syntax that can make code easier to read and write. Second, it can be more efficient for iterating over large collections or arrays, since it uses internal iteration rather than external iteration. Finally, the forEach method can be used with lambda expressions, allowing for more flexible and powerful code.
Conclusion
In conclusion, I hope this tutorial has provided a comprehensive understanding of one of the most fundamental concepts in Java programming. By mastering the syntax and usage of for loops, you can write efficient and effective code that can perform complex operations on arrays and collections. Additionally, we have explored the Java forEach method, which provides an alternative and concise way to iterate over arrays and collections.
By combining this knowledge with other Java programming concepts, you can take our Java programming skills to the next level and build powerful applications. I encourage you to continue practicing and experimenting with for loops to become a more proficient Java programmer. Don’t forget to check out the Java Tutorial for Beginners page for more Java tutorials.
Frequently asked questions
- What is difference between while loop and for loop?
Both the while loop and the for loop are used for iterating through a block of code, but they differ in syntax and usage. The while loop continues to execute the block of code as long as a specified condition is true, whereas the for loop is used for iterating through a known range of values or a known number of times. In a while loop, you must manually update the loop variable within the loop block, whereas in a for loop, the loop variable is automatically updated as part of the loop syntax. - What is the most common problem with the use of loops in programming?
The most common problem with the use of loops in programming is creating an infinite loop. An infinite loop occurs when the loop condition is never met, causing the loop to continue indefinitely. This can cause a program to become unresponsive and can even crash the system. It’s important to always ensure that the loop condition is properly defined and that there is a way for the loop to exit, such as through the use of a break statement or other conditional statements. - What is a real life example of for loops in programming?
A real-life example of for loops in programming could be when working with an inventory management system for a retail store. The program could use a for loop to iterate through each item in the store’s inventory, updating the stock levels or prices as needed. This would allow the program to efficiently manage a large number of items, reducing the need for manual data entry and ensuring that the inventory is always accurate and up-to-date. - How do I choose between using a for loop and a forEach loop?
You can choose between using a for loop and a forEach loop based on the type of collection or array you are iterating over, and your specific needs in terms of performance and readability. If you are working with an array or an object that implements the Iterable interface, the forEach loop can be a more concise and readable option, while a for loop may be more suitable for iterating over collections that don’t implement the Iterable interface. It’s also worth considering that the forEach loop uses internal iteration, which can be more efficient than external iteration used in for loops. Ultimately, the choice between the two will depend on the specific requirements of your code.