Java main method is the entry point of any Java program. It’s the method that the Java Virtual Machine (JVM) calls to execute the program.
In this tutorial, we’ll explore what the main method is, how it’s executed, and what the valid Java main method signatures are. We’ll also discuss some best practices for writing the main method, such as separating concerns, using appropriate design patterns, and writing testable code. Whether you’re new to Java programming or just need a refresher on the main method, this tutorial has got you covered.
What is the main method in Java?
The main method in Java is the entry point of any Java program. It is a method that must be included in the class that you want to run as a Java application. When the Java interpreter starts executing the application, it calls the class’s main method. The main method then calls all other methods needed to execute the application.
The main method has a specific signature: “public static void main(String[] args)”. This signature specifies the required format for the main method.
In the body of the main method, you typically place a call to other methods to initiate the execution of your code. Without such a call, the program will run, but the rest of the code will not be executed. Here’s an example:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
In this example, the main method simply prints the message “Hello, World!” to the console using the System.out.println()
method.
Let’s break down each component of the signature mentioned above:
public
: This is an access modifier in Java that indicates the visibility of the method.public
means that the method can be accessed from anywhere in the program.static
: This is a keyword in Java that indicates that the method belongs to the class rather than an instance of the class. In other words, you can call themain
method without creating an object of the class that contains it.void
: This is the return type of themain
method, which means that the method does not return any value.main
: This is the name of the method. It is a reserved name in Java and must be used exactly as shown.String[] args
: This is a parameter of themain
method. It represents the command-line arguments that can be passed to the program when it is executed. Theargs
parameter is an array of strings, where each string represents a single command-line argument.
It’s important to note that the main
method must accept exactly one parameter, which is an array of strings. You can change the name of the args
parameter to whatever you like, but the type of the parameter must remain String[]
.
Overall, the main
method is a crucial part of any Java program as it serves as the starting point of the program’s execution.
Execution of the main method
Let’s write a simple Java program that demonstrates the main
method. Open your favorite text editor and type the following code:
public class App { public static void main(String[] args) { for(String s : args) { System.out.println(s); } } }
Save this file as App.java
.
Here, we have defined a class named App
that contains the main
method. Inside the main
method, we have a for-loop that will go through the elements of the args
array and print them using the System.out.println()
method. The args
array contains the command-line arguments passed to the program when it is executed.
Compiling and Executing the Program
To run the program, we need to compile the App.java
file and then execute the compiled program. Follow these steps:
- Open your command prompt or terminal window.
- Navigate to the location where you saved the
App.java
file. - Compile the
App.java
file by running the command:javac App.java
. This will create a compiled version of theApp.java
file calledApp.class
. - Execute the compiled program by running the command:
java App
. This will run themain
method of theApp
class and print the elements of theargs
array to the console.
If you have followed these steps correctly, the expected output should be displayed:
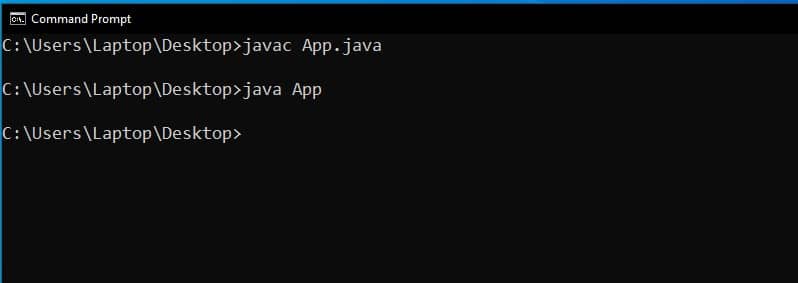
If you didn’t see any output after executing the program, it is because we didn’t pass any parameters to the main method for it to print.
To see some output, let’s execute the code again by passing the word “Hello” as a parameter. Type “java App Hello” in the command prompt and hit enter. You should now see the word “Hello” printed as the program output.
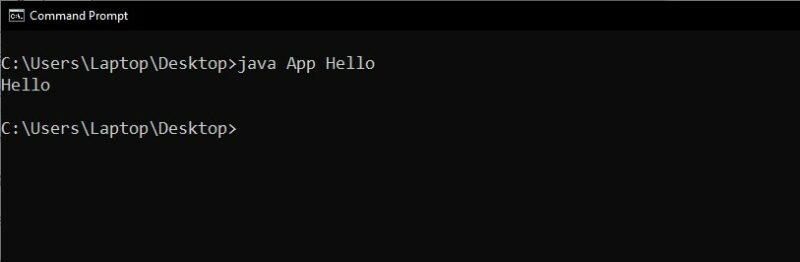
Given that the parameter of the main method is an array of strings, we can pass multiple arguments as parameters when executing the program. For example, running the command ‘java App Hello World!’ would result in the following output:
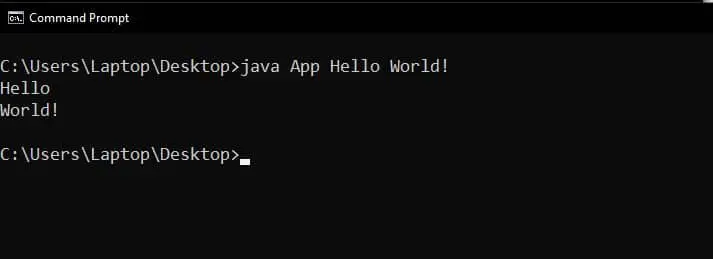
Regardless of the number of words passed, the Java for-loop iterates through each element of the args array and prints it, as demonstrated.
That’s it! You have now successfully compiled and executed a simple Java program that demonstrates the use of the main
method.
Valid Java main Method Signature
The Java main method is the method that the Java Virtual Machine (JVM) looks for when starting a Java program. It must be defined with a specific signature to be recognized by the JVM.
The valid Java main method signatures are:
public static void main(String[] args)
public static void main(String []args)
public static void main(String args[])
public static void main(String... args)
static public void main(String[] args)
public static final void main(String[] args)
final public static void main(String[] args)
All of these signatures are functionally equivalent and can be used interchangeably. However, it is important to note that any deviation from these signatures will result in the JVM being unable to recognize the method as the entry point for the Java program.
It is also worth noting that the name of the Java main method must be “main”. Any deviation from this name will also result in the JVM being unable to recognize the method as the entry point for the Java program.
In addition to the method signature and name, the Java main method must also be defined within a class. The class can have any name, but it must contain the Java main method.
It is good practice to include a comment in the Java main method to explain the purpose of the program and any command-line arguments that are expected.
Here is another example of a Java main method that follows one of the valid signatures:
public class Calculator { public static void main(String[] args) { // This program calculates the sum of two numbers provided as command-line arguments if (args.length < 2) { System.out.println("Usage: java Calculator <num1> <num2>"); return; } int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); int sum = num1 + num2; System.out.println("The sum of " + num1 + " and " + num2 + " is " + sum); } }
In this example, the Calculator
class contains a main
method that takes two command-line arguments and calculates their sum. The program checks that there are at least two command-line arguments provided and prints an error message if there are not. It then parses the two arguments as integers, calculates their sum, and prints the result to the console. The comment in the main
method explains the purpose of the program and the expected usage.
Best practices for writing the main method
While the main method can contain any valid Java code, there are some best practices that can help make the code more readable, maintainable, and testable. Adhering to these best practices can also help avoid common pitfalls and make the main method easier to understand and debug.
Separate concerns
One of the most important best practices for writing the main method is to separate concerns. This means dividing the code into smaller, more manageable pieces that are responsible for distinct tasks. By doing this, you can make the code more modular, easier to read, and easier to test.
Here are some examples of how you can separate concerns in the main method:
-
Delegate complex logic to other methods or classes: If the main method contains complex logic or functionality, consider extracting it into a separate method or class. This can help make the code more readable and maintainable, and can also make it easier to test the logic in isolation.
-
Minimize side effects: Try to avoid writing to standard output or changing the state of global variables from within the main method. Instead, pass any necessary parameters to other methods or classes, and let them handle the side effects.
-
Handle exceptions gracefully: If the main method can throw exceptions, make sure to catch them and handle them gracefully. This can involve logging errors, displaying user-friendly error messages, or exiting the program with a non-zero exit code.
Use appropriate design patterns
Another best practice for writing the main method is to use appropriate design patterns. Design patterns are proven solutions to common programming problems, and can help make the code more modular, reusable, and maintainable.
Here are some examples of design patterns that can be useful in the main method:
-
Factory method pattern: If the main method needs to create instances of different classes based on some condition, consider using the factory method pattern. This involves defining a separate factory class or method that is responsible for creating instances of the appropriate class.
-
Singleton pattern: If the main method needs to access a shared resource (e.g. a database connection), consider using the singleton pattern. This involves defining a single instance of a class that provides access to the shared resource.
-
Strategy pattern: If the main method needs to perform different algorithms or actions based on some condition, consider using the strategy pattern. This involves defining separate classes or methods that implement the different algorithms or actions, and passing them as parameters to the main method or other classes.
Write testable code
Finally, a best practice for writing the main method is to write testable code. Writing testable code means writing code that can be easily tested using automated tests, such as unit tests or integration tests.
Here are some tips for writing testable code:
-
Use dependency injection: Instead of creating instances of other classes directly within the main method, consider using dependency injection to inject them as parameters. This can make it easier to write unit tests for the main method, as you can replace the injected dependencies with mock objects or test doubles.
-
Extract logic into separate methods or classes: If the main method contains complex logic, consider extracting it into a separate method or class. This can make it easier to write unit tests for the logic in isolation, without having to test the entire main method.
-
Write clear and concise code: Finally, make sure to write clear and concise code that is easy to understand and maintain. This can help avoid errors and make it easier to write effective tests.
By following these best practices, you can write main methods that are more readable, maintainable, and testable, and that are less likely to contain errors or cause problems down the line.
Conclusion
In this tutorial, we covered the basics of Java main method, its role in Java programming, how it’s executed, and what the valid Java main method signatures are. We also discussed some best practices for writing the main method, including separating concerns, using appropriate design patterns, and writing testable code. With this knowledge, you’ll be able to write better Java programs that are well-organized, efficient, and maintainable. Remember to keep practicing and learning to become a skilled Java developer.
Frequently asked questions
- Is it mandatory to have the main method in a Java program?
Yes, it is mandatory to have the main method in a Java program. The main method serves as the entry point of the Java program and is required by the Java Virtual Machine (JVM) to execute the program. If a Java program doesn’t have a main method, the JVM won’t be able to execute it, and an error will occur. - Can we have multiple main methods in a Java program?
No, we cannot have multiple main methods in a Java program. Each Java program must have only one main method, which serves as the entry point of the program. If we define more than one main method in a Java program, the compiler will not know which method to execute when we run the program, and an error will occur. - What happens if we define the main method with a return type other than void?
If we define the main method with a return type other than void, the Java compiler will generate a compilation error. This is because the JVM looks for a method with a specific signature, which includes a public static void main method, to execute the Java program. Changing the return type of the main method would violate this requirement, and thus the program would not compile. - Can we overload the main method in Java?
Yes, we can overload the main method in Java. Overloading is a concept in Java where we can define multiple methods with the same name in a class, but with different parameters. When we overload the main method in Java, we define multiple main methods with different parameter lists. However, only the main method with the standard signature (public static void main(String[] args)) is called when we run the Java program. The overloaded main methods can be called from within the program like any other method with the same name.